Legacy Java Build Systems: A Survival Guide
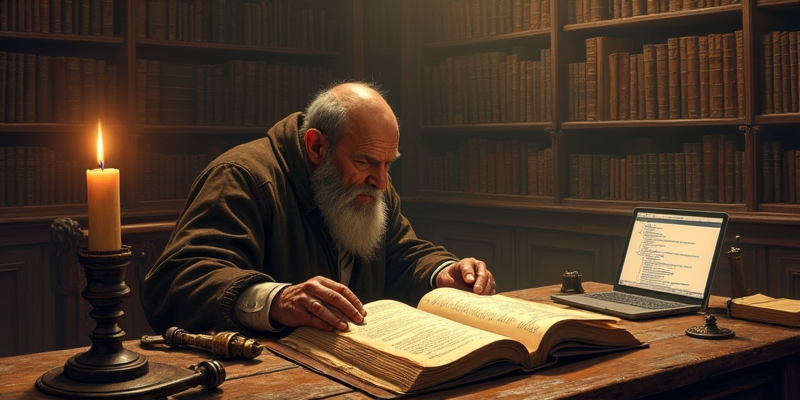
Introduction
Maintaining legacy Java build systems doesn’t have to be a nightmare. Whether you’re stuck with Ant, Maven 1, or an early version of Gradle, this guide provides actionable steps to keep your builds running smoothly while paving the way for modernization. Let’s dive into practical strategies you can implement today.
Step 1: Document the Build Process
Start by creating a clear, up-to-date record of how your build system works. This will save you (and your team) countless hours of frustration.
What to Document:
- Build Scripts: Open your
build.xml
(Ant) orpom.xml
(Maven) and add comments to explain each target or task. For example:
<!-- Compiles all source files in the src directory -->
<target name="compile">
<javac srcdir="src" destdir="build/classes"/>
</target>
-
Dependencies: List all dependencies, including their versions and sources. Use a spreadsheet or a simple text file.
-
Build Steps: Write down the exact commands and environment setup needed to run the build. For example:
# Set Java home to JDK 1.8
export JAVA_HOME=/usr/lib/jvm/java-8-openjdk
# Run the Ant build
ant compile
Pro Tip:
Use tools like Doxygen or MkDocs to generate searchable, user-friendly documentation.
Step 2: Modernize Dependency Management
Legacy systems often rely on outdated or hard-to-find dependencies. Here’s how to fix that:
For Ant Projects:
- Use Ivy: Integrate Apache Ivy to manage dependencies. Add an
ivy.xml
file to declare dependencies:
<dependencies>
<dependency org="junit" name="junit" rev="4.12"/>
</dependencies>
Then, update your build.xml
to resolve dependencies using Ivy.
For Maven 1 Projects:
- Migrate to Maven 3: Create a new
pom.xml
for Maven 3 and gradually move your dependencies. Use themvn dependency:tree
command to analyze your current setup.
For All Projects:
- Host Dependencies Locally: Set up a private repository using tools like Nexus or Artifactory to ensure dependencies are always available.
Step 3: Automate Repetitive Tasks
Legacy build systems often require manual steps. Automate them to save time and reduce errors.
Using Make and Shell Scripts
Make
and shell scripts are lightweight, portable, and easy to set up in most environments. They make the build process transparent and maintainable.
Basic Concepts:
- Makefile: A
Makefile
defines rules for building targets. Each rule specifies a target, its dependencies, and the commands to execute. - .PHONY: Use
.PHONY
to declare targets that aren’t files (e.g.,clean
orbuild
). This prevents conflicts with files of the same name.
Example Makefile:
# Declare phony targets
.PHONY: clean compile test package
# Compile Java source files
compile:
javac -d build/classes src/*.java
# Run tests
test: compile
java -cp build/classes org.junit.runner.JUnitCore MyTest
# Package the application
package: compile
jar cf build/myapp.jar -C build/classes .
# Clean build artifacts
clean:
rm -rf build/*
Example Shell Script:
#!/bin/bash
# Set Java home to JDK 1.8
export JAVA_HOME=/usr/lib/jvm/java-8-openjdk
# Compile source files
javac -d build/classes src/*.java
# Run tests
java -cp build/classes org.junit.runner.JUnitCore MyTest
# Package the application
jar cf build/myapp.jar -C build/classes .
Why Use Make and Shell Scripts?
- Transparency: The build process is clearly defined in the
Makefile
or script, making it easy for future maintainers to understand. - Portability: These tools are available on almost all Unix-like systems and can be easily adapted for Windows.
- Flexibility: You can integrate them with other tools like Ant, Maven, or Gradle for a hybrid approach.
Step 4: Gradually Modernize the Build System
Instead of rewriting everything at once, take a phased approach.
For Ant Projects:
- Introduce Gradle: Start by converting a single module to Gradle. Use the
ant.importBuild
method to integrate existing Ant tasks:
ant.importBuild 'build.xml'
- Use Gradle Plugins: Leverage plugins for dependency management, testing, and packaging.
For Maven 1 Projects:
- Migrate to Maven 3: Create a new
pom.xml
and move dependencies one at a time. Use themvn help:effective-pom
command to understand your current configuration.
For Early Gradle Projects:
- Upgrade Gradle: Update to the latest Gradle version and use the
gradle wrapper
to ensure consistency.
Step 5: Test and Validate
Before fully switching to a new build system, ensure it produces the same output as the legacy system.
How to Test:
- Compare Build Artifacts: Run both the legacy and new build systems and compare the resulting JARs or WARs.
- Automate Testing: Write integration tests to verify the build output. For example, use JUnit to test the functionality of the built artifact.
Step 6: Plan for the Future
Once your build system is stable, plan for long-term maintenance:
- Schedule Regular Reviews: Periodically review and update your build scripts and dependencies.
- Train Your Team: Ensure everyone understands the new build process and tools.
- Monitor Build Performance: Use tools like Gradle Build Scan or Jenkins metrics to identify bottlenecks.
Conclusion
Legacy Java build systems don’t have to hold you back. By documenting your build process, modernizing dependency management, automating repetitive tasks with tools like Make
and shell scripts, and gradually introducing modern tools, you can keep your projects running smoothly while preparing for the future.